All projects are listed in order of completion, with the newest at the top and the oldest at the bottom for each category.
Personal Projects
Resume
html css web-design
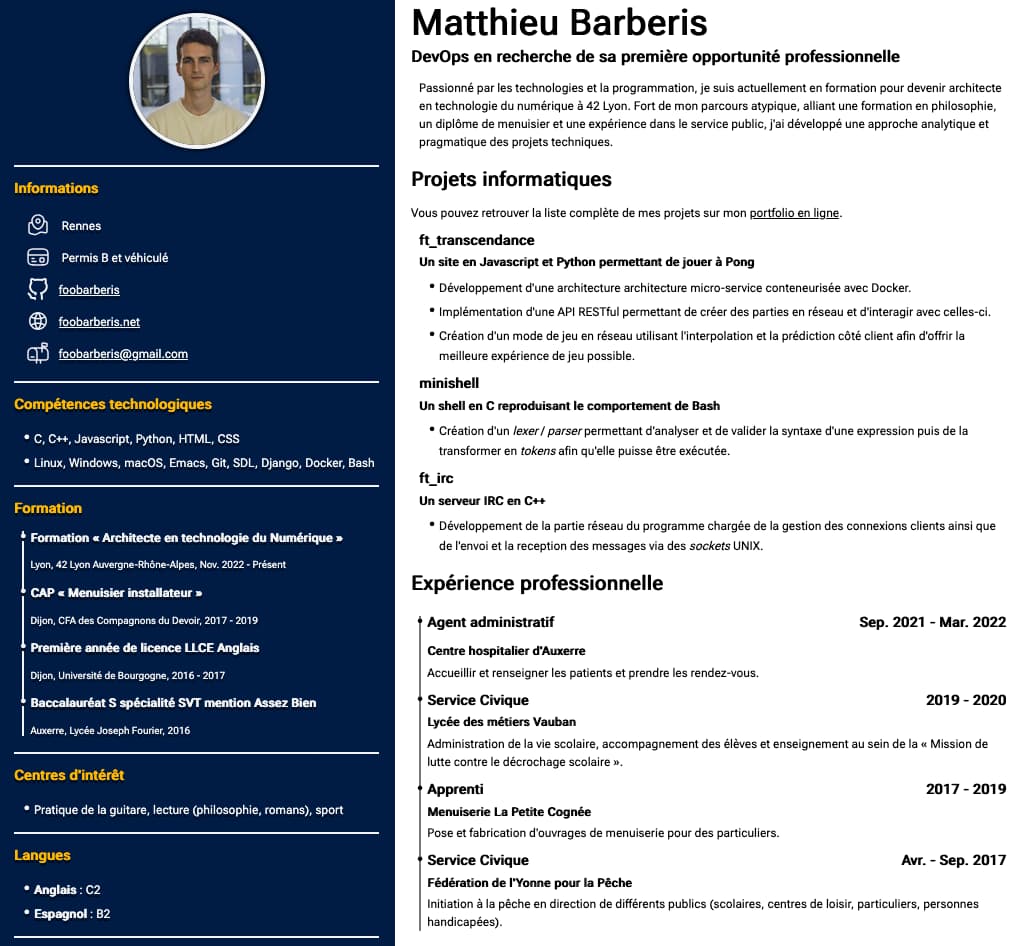
My personal resume made from scratch with HTML and CSS. It is designed to look good both as a webpage and as a PDF.
- Custom-coded with pure HTML and CSS.
- Used Tachyons CSS for atomic styling and rapid development.
- Fully responsive design for all screen sizes.
- Designed to be both visually appealing and print-friendly on a single A4 PDF.
- Optimized for printing, ensuring the layout remains clean and professional when converted to a PDF.
Truff' and Co
html css web-design
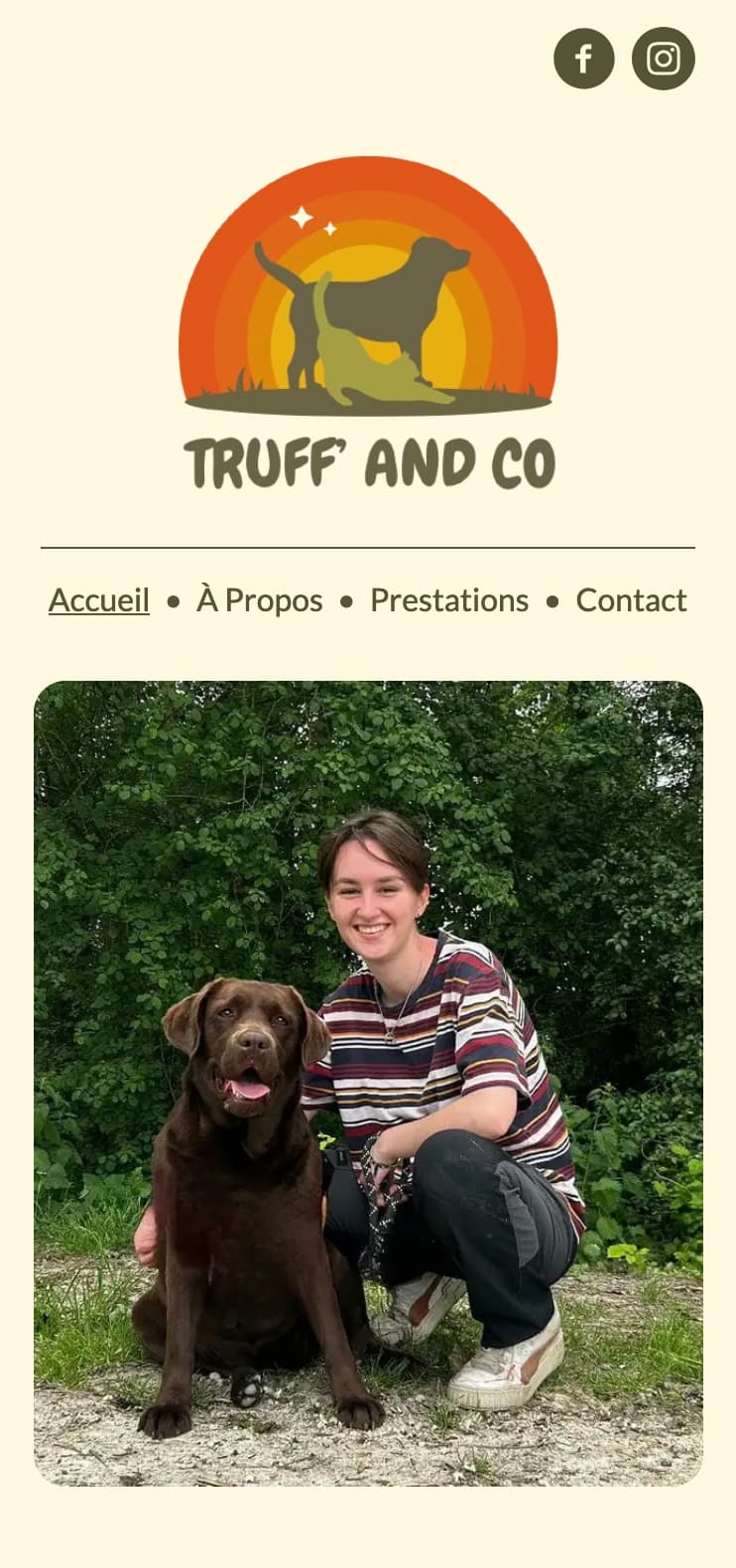
A website I developed for my sister's pet-sitting business. It is designed to be simple and lightweight, yet visually appealing.
- Custom-coded with pure HTML and CSS.
- Used Tachyons CSS for atomic styling and rapid development.
- Fully responsive design for all screen sizes.
- Designed with accessibility in mind, meeting high accessibility standards.
- Lightweight and fast (entire site is just 87KB excluding images).
3D Computer Graphics Programming
3d-graphics c mathematics
A course on 3D graphics programming using C, covering key concepts like transformations, projections, and lighting.
- Gained an understanding of core 3D graphics principles like camera transformations, projection matrices, and basic lighting models.
- Learned to implement C programming techniques for rendering 3D objects, including algorithms for rasterization and texture mapping.
- Worked hands-on with key math concepts such as vectors, matrices, and coordinate systems.
Atari 2600 Programming with 6502 Assembly
computer-architecture 6502-assembly
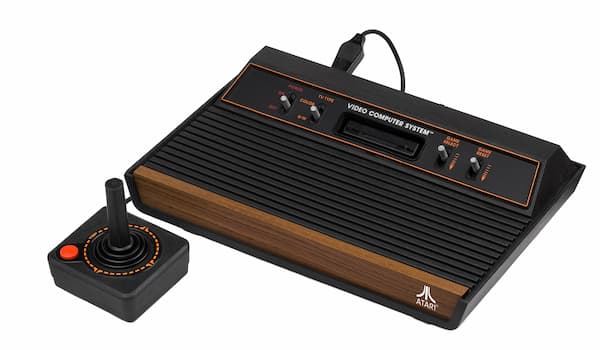
A course on 6502 assembly language and computer architecture, focusing on programming games for the Atari 2600 console.
- Learned the basics of 6502 assembly and low-level programming.
- Gained a solid understanding of the Atari 2600 hardware and memory map.
- Developed a homebrew game for the Atari 2600 from scratch.
42 School Projects
You can find all my projects for 42 in this repository or you can click on each project's name to go directly to the relevant code.
ft_transcendence
css django docker git html javascript nginx python teamwork
A website that allows users to play Pong locally, with additional features like login, user profiles, and online play.
- Containerized microservice architecture using Docker.
- Game graphics, physics, and sound effects implemented in pure JavaScript.
- Remote play using client-side prediction and interpolation for a smooth experience.
- Option to play against other users locally, remotely, or against the CPU.
- Login system for a personalized user experience, granting access to user profiles, friend lists, and match and tournament history.
ft_irc
c++ git network-programming teamwork
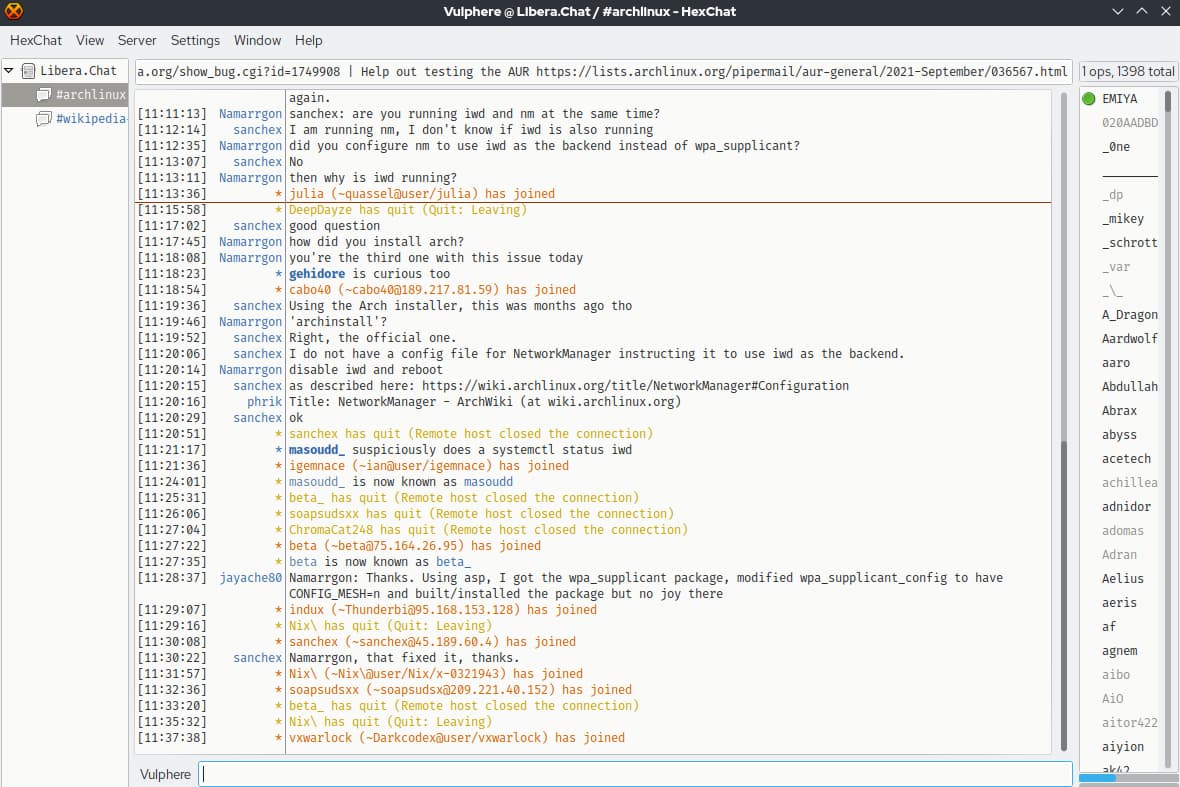
An IRC server compliant with RFC 1459, capable of handling multiple simultaneous connections.
- Compliance with RFC 1459 standards for the IRC protocol.
- Designed to handle many simultaneous connections without blocking or forking.
- Network code developed using the Linux socket interface.
- Implements all essential IRC commands to manage users and channels.
C++ Modules
c++ git object-oriented-programming
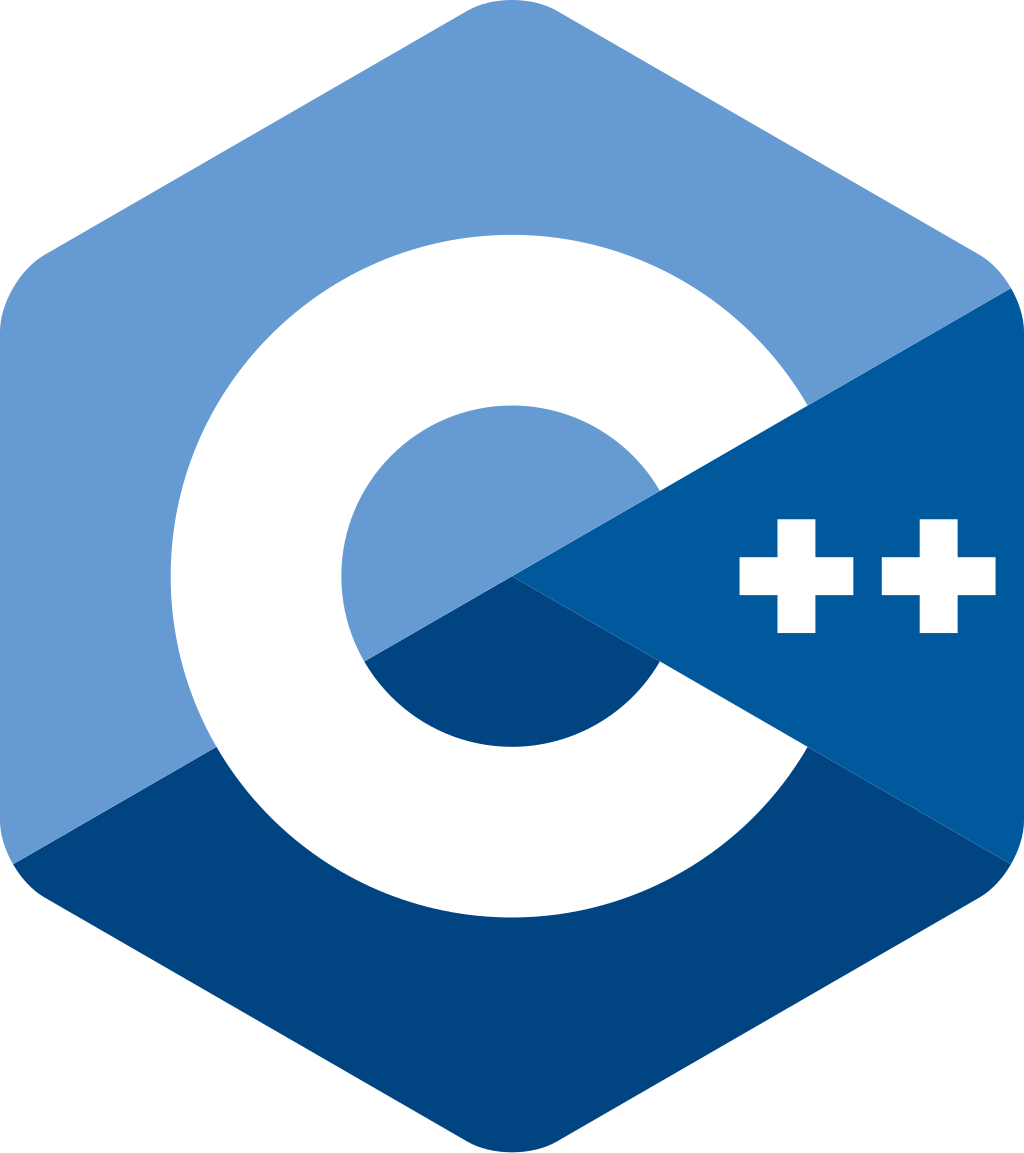
An introduction to object-oriented programming and C++, covering the core features of C++98.
- Covers essential features of C++98 such as polymorphism, templates, and the STL.
- Provides a foundation for learning more modern versions of C++.
- Includes various exercises to reinforce key C++ concepts.
cub3d
c git teamwork graphics-programming
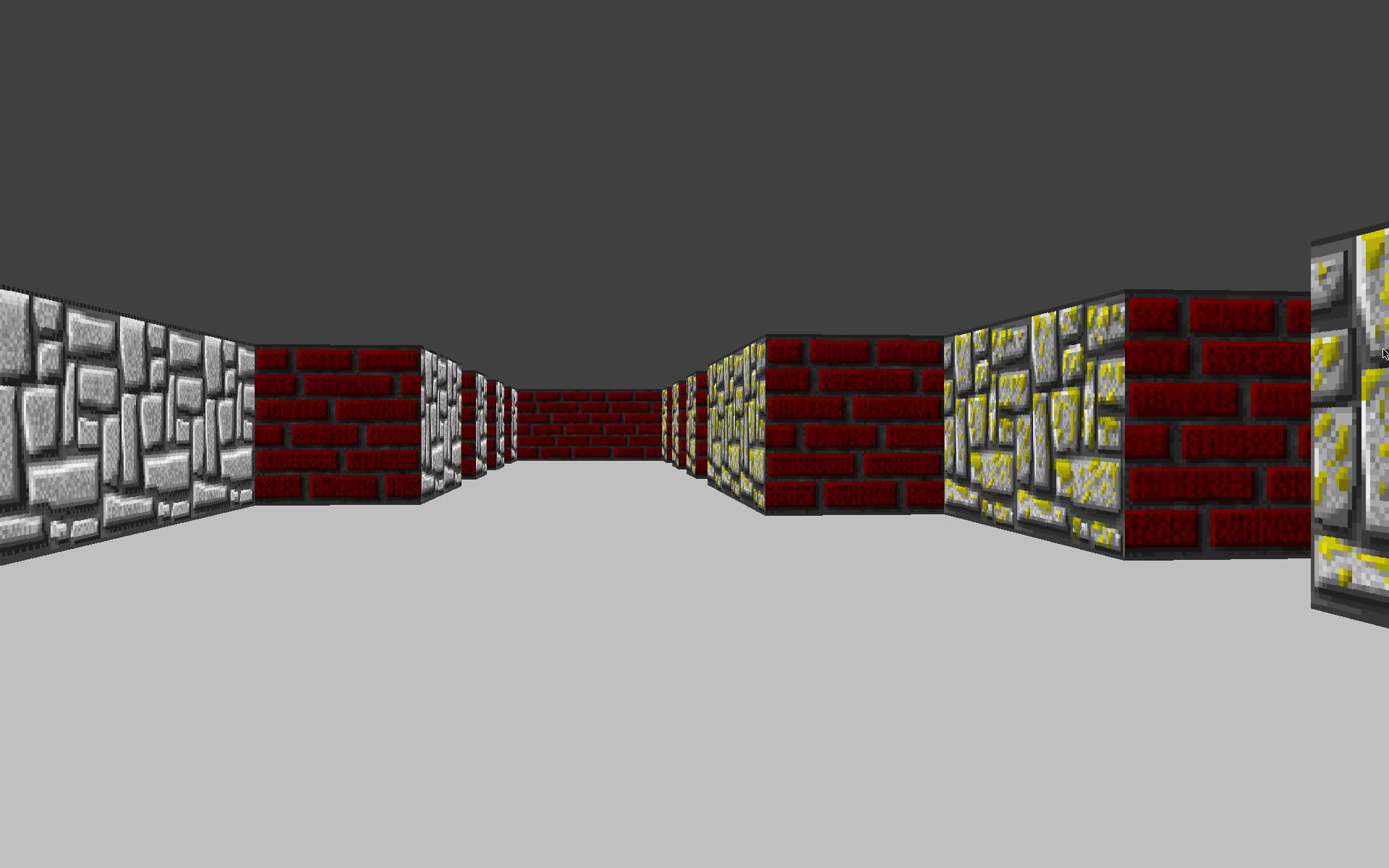
A game similar to Wolfenstein 3D, using ray casting, providing an introduction to computer graphics.
- Developed a 3D game using ray casting, similar to Wolfenstein 3D.
- Implemented the ability to create, modify, and share maps via a simple text-based format, allowing users to change the layout and textures of the map.
- Introduced to computer graphics and 3D rendering techniques.
- Learned the geometry behind transforming 2D images into 3D visuals.
- Gained hands-on experience with fundamental graphics algorithms and techniques.
inception
docker mariadb networking nginx wordpress
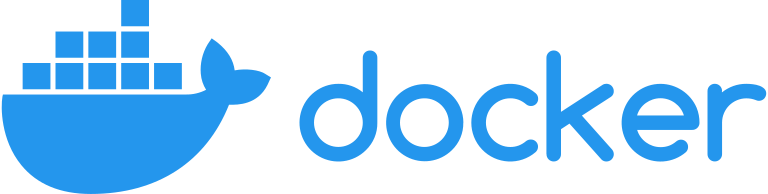
A WordPress website using Docker, offering an introduction to virtualization and containerization.
- Created a WordPress website using Docker and Docker Compose.
- Built all Docker images from scratch rather than using pre-made ones.
- Configured MariaDB to work seamlessly with WordPress.
- Set up Nginx as a reverse proxy to enhance performance.
- Deepened understanding of Docker and container orchestration.
- Gained hands-on experience with Docker and virtualization concepts.
netpractice
networking
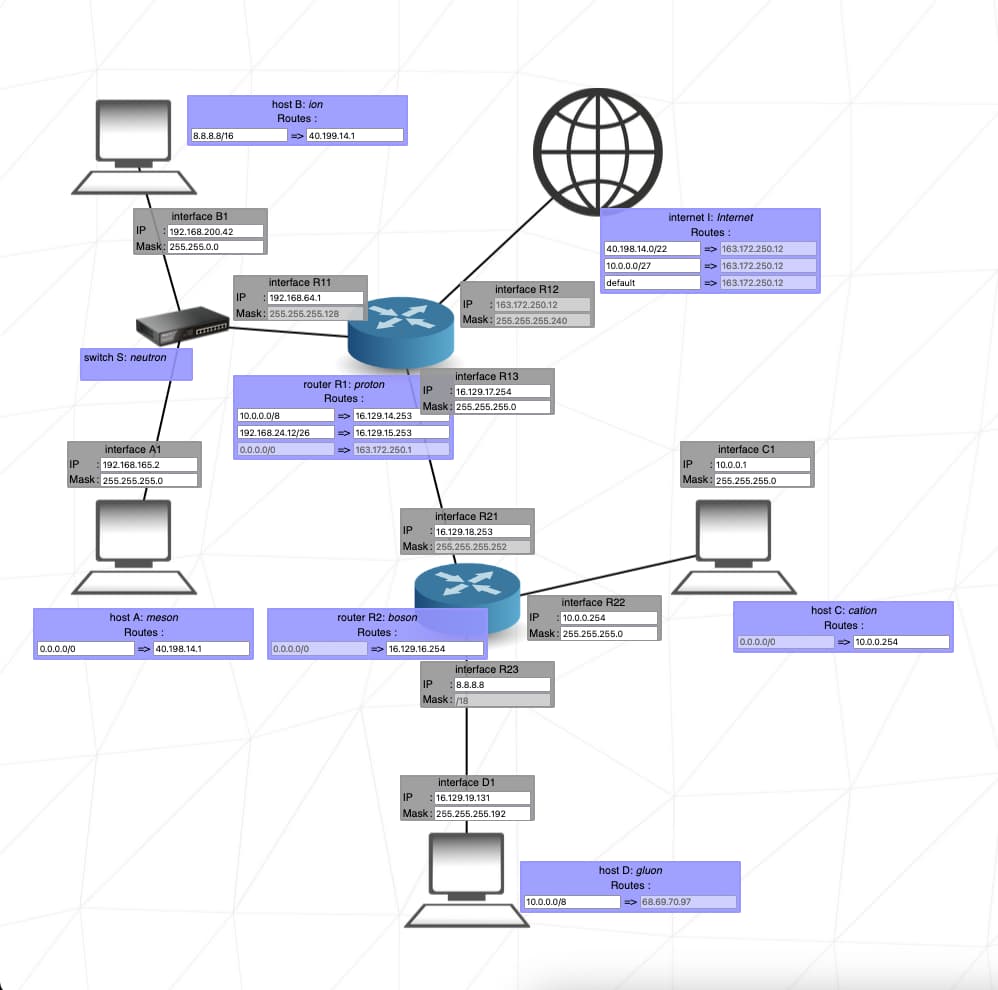
This project focuses on learning about networking and TCP/IP by configuring small-scale networks through puzzles.
- Focused on networking fundamentals and TCP/IP protocols.
- Learned practical network configuration through a series of puzzles.
- Gained hands-on experience with small-scale network setups.
- Developed problem-solving skills in networking scenarios.
minishell
c git shell system-programming teamwork
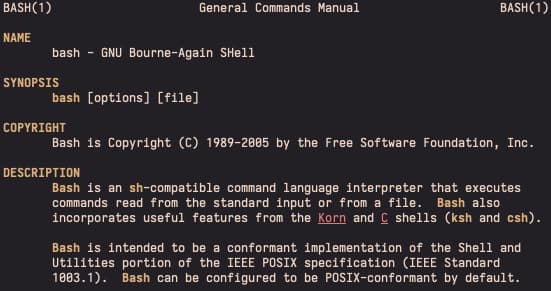
A simplified version of Bash, supporting pipes, redirections, and tilde expansion.
- Created a simplified version of Bash, implementing key shell features.
- Supported pipes (|), redirections (>, <, >>), and tilde (~) expansion.
- Implemented the lexer and parser to process user input into executable tokens.
- Gained hands-on experience with shell internals and command-line tools.
philosophers
c git multithreading system-programming
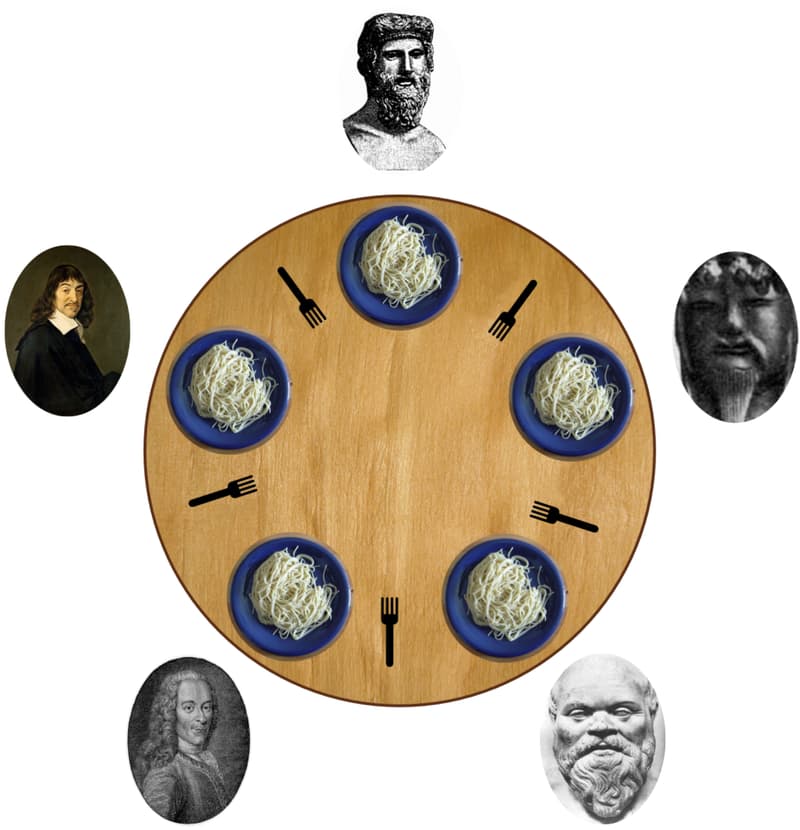
This project introduces multithreading and synchronization using mutexes, specifically addressing the dining philosophers problem.
- Introduced to multithreading and synchronization concepts.
- Solved the classic dining philosophers problem using mutexes.
- Implemented argument handling for the number of philosophers, eating/sleeping times, and starvation limits.
- Gained hands-on experience with thread synchronization in a real-world problem.
- Learned to manage resources and prevent deadlocks in concurrent programs.
fdf
c git graphics-programming
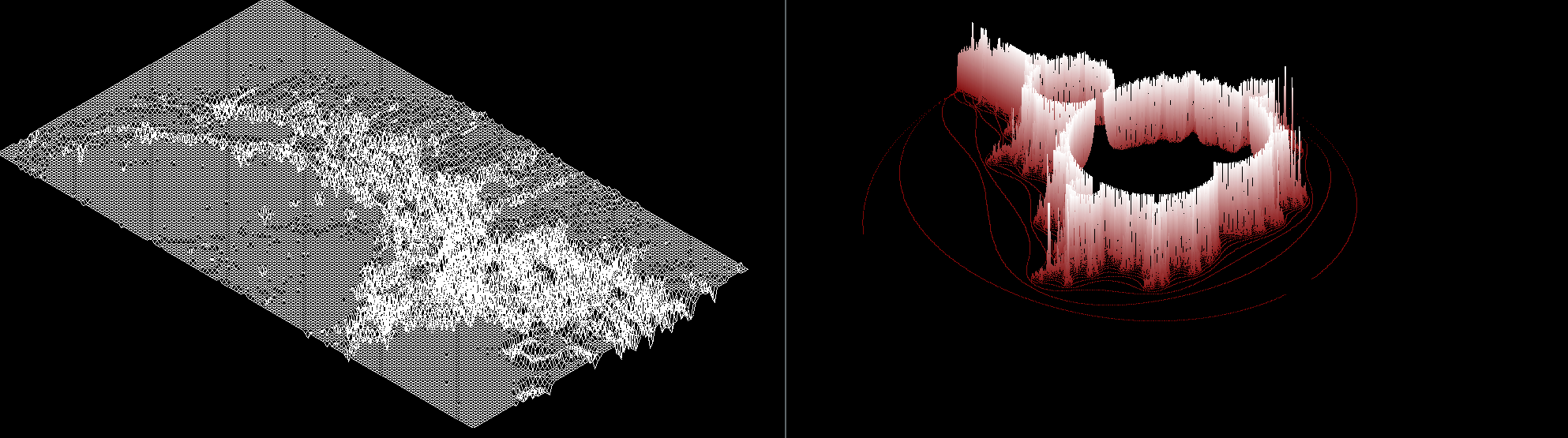
This project focuses on representing a landscape using wireframe graphics, processing data from a text file to create a visual representation.
- Developed a wireframe representation of a landscape using data from a text file.
- Validated text file input to ensure data integrity.
- Processed elevation data to render an accurate 3D landscape view.
- Gained hands-on experience with file parsing and graphical rendering techniques.
- Learned the basics of 3D graphics representation using wireframe models.
push_swap
c git algorithms
This project introduces algorithms and computational complexity by sorting data on a stack using the fewest possible actions.
- Introduced to algorithms and computational complexity.
- Sorted data on a stack with a restricted set of instructions.
- Optimized the sorting solution to minimize the number of actions.
- Gained hands-on experience with stack-based data manipulation and problem-solving.
- Developed a deeper understanding of algorithm efficiency and optimization.
minitalk
c git signals system-programming
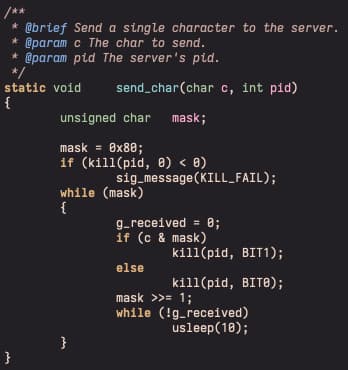
A small data exchange program using UNIX signals, with added support for Unicode characters and acknowledgement mechanisms.
- Implemented a data exchange program using UNIX signals.
- Supported Unicode characters for enhanced message compatibility.
- Included an acknowledgement mechanism for server-client communication.
- Ensured reliable message delivery with feedback from the server.
- Gained experience with UNIX signals and inter-process communication.
get_next_line
c git system-programming
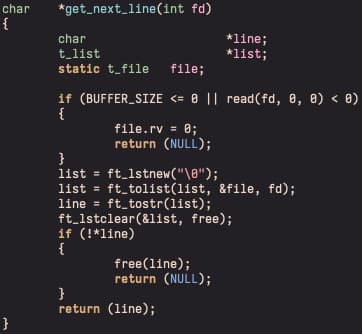
A function similar to getline(3) that reads an input stream line by line.
- Created a function to read input streams line by line.
- Worked with pointers and null-terminated strings for memory management.
- Gained hands-on experience with string handling and buffer management.
- Strengthened understanding of low-level input/output operations in C.
born2beroot
devops cybersecurity linux shell
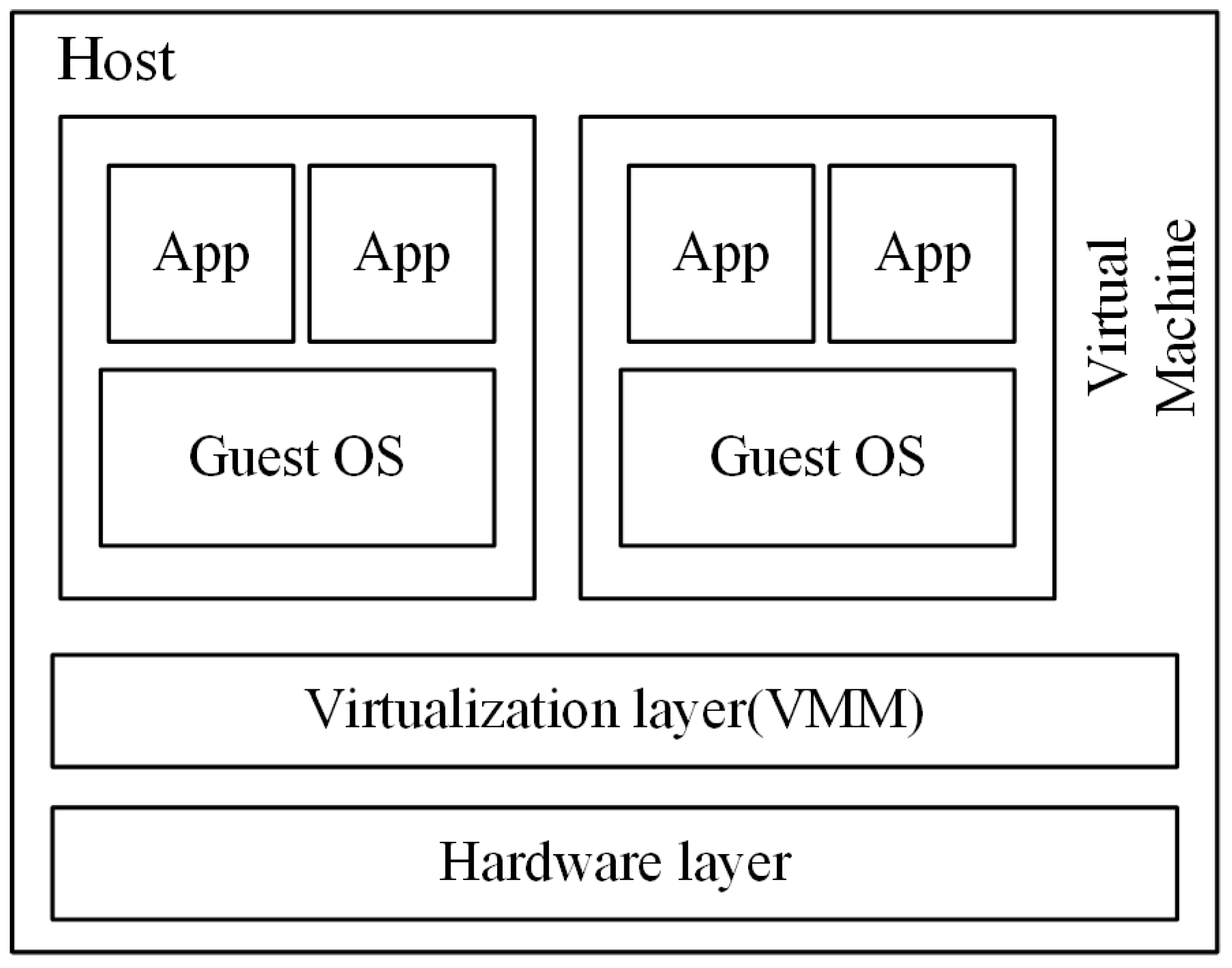
This project involves installing, configuring, and monitoring a Linux virtual machine, with a focus on security and system management.
- Installed and configured a Linux virtual machine.
- Created partitions using Logical Volume Manager (LVM).
- Set up and managed a firewall using UFW.
- Configured AppArmor for enhanced system security.
- Implemented a strong password policy using Pluggable Authentication Modules (PAM).
- Gained hands-on experience with Linux system administration and security practices.
ft_printf
c git system-programming
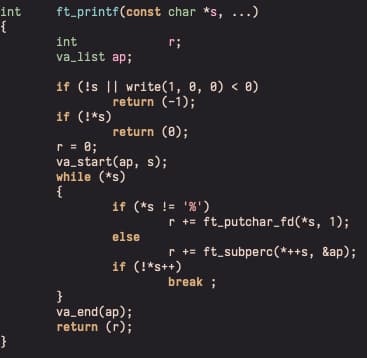
A reimplementation of the C standard library function printf(3) to handle multiple format conversions.
- Handled format conversions: c, s, p, d, i, u, x, X, and %.
- Worked with variadic functions to process variable argument lists.
- Gained understanding of how to handle different data types in formatted output.
- Enhanced skills in memory management and function argument handling.
libft
c git system-programming
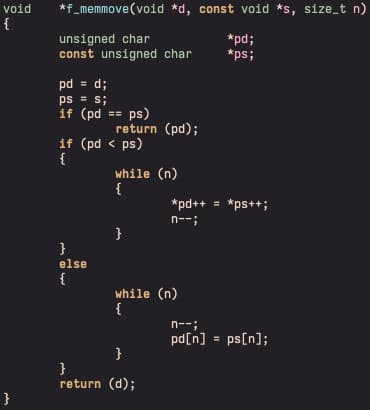
A C library containing general-purpose functions, focusing on improving utility and optimization for future programs.
- Developed a C library with various general-purpose functions.
- Included functions for linked list handling, tailored to specific structs.
- Identified and replaced inefficient or redundant functions, such as replacing `bzero` with `memset`.
- Created mlc, a more efficient, optimized version inspired by musl libc.
- Focused on providing a leaner, more useful set of functions for future projects.
- Improved understanding of C library design and optimization strategies.
42 Piscine
c git problem-solving teamwork
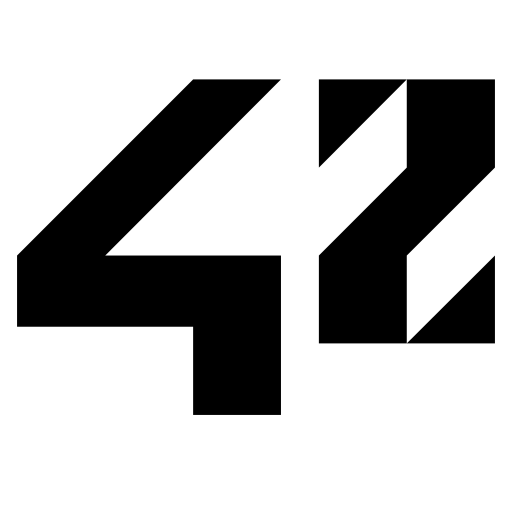
This repository contains all the exercises I completed during the Piscine (the one-month recruitment process for 42).
- Completed various exercises as part of the 42 recruitment process (Piscine).
- Showcases a range of tasks that demonstrate problem-solving and coding skills.
- Gained foundational knowledge and hands-on experience in C programming.
- Serves as a portfolio of work completed during the intense one-month selection period.